codebeamer Development
The following pages contain information about development opportunities and processes with codebeamer, such as:
- codebeamer API-reference, -extensions and plugin development,
- Wiki plugin development,
- Jenkins plugin development,
- Word template development for codebeamer, etc.
Some pages contain procedural information, while others only refer to the pages containing documnetation of the related developer tools of the processes in question.
codeBeamer Developer's Guide
This manual is intended for developers and describes concepts that you need to understand in order to use codeBeamer Web APIs to extend it using its various extension points.
codeBeamer is a Spring
based Java
application, running as a Web Service
in an Apache Tomcat®
container.
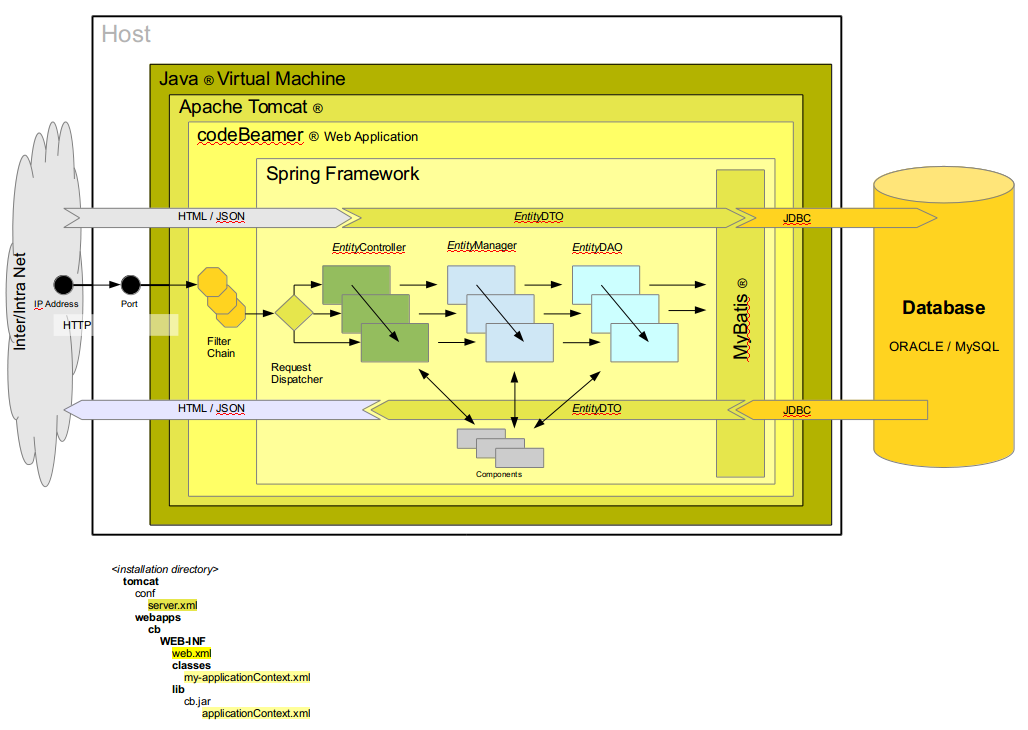
The Apache Tomcat®
provides the container for the codeBeamer (cb) web application and is responsible for
- Accepting and handling incoming HTTP requests
- Providing threads for the request processing
- Routing the requests to the codeBeamer web application via the appropriate filters.
The tomcat server configuration, e.g.
- Listen ports,
- Request protocols,
- Thread pool parameters
are defined in /tomcat/conf/server.xml in the codeBeamer installation directory.
The request routing and filter configuration for the codeBeamer (cb) Web Application
is defined in /tomcat/webapps/cb/WEB-INF/web.xml in the codeBeamer installation directory.
The context
for the codeBeamer application is provided by the Spring Framework
, not via Tomcat:
- Bean instantiation and lifecycle management
- Application startup and shutdown
- Dependency injection
- Transaction management
- Internal request routing according to the path of the request URL
to the appropriate controllers
The standard application context for codeBeamer is defined via applicationContext.xml in /tomcat/webapps/cb/WEB-INF/lib/cb.jar.
Customer specific application context enhancements can be defined via my-applicationContext.xml in /tomcat/webapps/cb/WEB-INF/classes.
To add custom beans, e.g.
Listeners or
Workflow Actions:
- Add the compiled bean classes to /tomcat/webapps/cb/WEB-INF/classes.
- Or, if the beans are packed into a JAR
, add the JAR to /tomcat/webapps/cb/WEB-INF/lib.
Depending on the type of bean, you may also have to deploy additional resources, e.g.
- Custom resource
localizations via my-ApplicationResources[locale].properties in /tomcat/webapps/cb/WEB-INF/classes
- Velocity
template files have to be added to the appropriate /tomcat/webapps/cb/config/templates sub-directory, e.g
- /email - for email subject and body templates
- /wiki-plugin - for Wiki plugin templates
- /widget - for dashboard widget templates
In order for custom beans (e.g.
Listeners or
Workflow Actions) to be recognized and used by the Spring Framework
- a <bean> configuration
must be added to /tomcat/webapps/cb/WEB-INF/classes/my-applicationContext.xml
- or (preferred), it will be automatically detected by the Component
scan during CodeBeamer startup, if
Standard codeBeamer Bean types
Controllers
Controllers are the HTTP
Request end-points.
They are responsible for validating and handling incoming requests and producing an appropriate response.
Depending on the type of controller or end-point, the request/response body can contain HTML
or JSON
.
Controllers should be annotated as @Controller and each HTTP
end-points as @RequestMapping, where the value is the path in the request URL
relative to the web application context path (/cb).
For example: The definition of the TrackerController and it's
Legacy REST API (v1) end-points for
- POST http://{server}:{port}/cb/tracker
- GET http://{server}:{port}/cb/tracker/{trackerId}
[{ColorCode syntax='java'
package com.intland.codebeamer.controller;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseStatus;
import com.intland.codebeamer.controller.ControllerUtils;
import com.intland.codebeamer.controller.support.ResponseView;
import com.intland.codebeamer.controller.support.RequestView;
import com.intland.codebeamer.manager.TrackerManager;
import com.intland.codebeamer.persistence.dto.TrackerDto;
import com.intland.codebeamer.persistence.dto.UserDto;
@Controller("trackerController")
public class TrackerController {
@Autowired
private TrackerManager trackerManager;
@RequestMapping(value = "/tracker", method = RequestMethod.POST)
@ResponseStatus(HttpStatus.CREATED)
@ResponseView
public TrackerDto createTracker(HttpServletRequest request, @RequestView("TrackerDetails") TrackerDto tracker)
throws AccessRightsException, ChangeVetoedException, IllegalArgumentException, ArtifactNameConflictException {
UserDto user = ControllerUtils.getCurrentUser(request);
trackerManager.create(user, tracker, request);
return tracker;
}
@RequestMapping(value = "/tracker/{trackerId}", method = RequestMethod.GET)
@ResponseView("TrackerDetails")
public TrackerDto getTracker(HttpServletRequest request, @PathVariable("trackerId") Integer trackerId) {
UserDto user = ControllerUtils.getCurrentUser(request);
TrackerDto tracker = trackerManager.findById(user, trackerId);
if (tracker == null) {
throw new ResourceNotFoundException("/tracker/" + trackerId);
}
return tracker;
}
@RequestMapping(value = "/proj/tracker/workflowGraph.spr", method = RequestMethod.GET)
public String displayTransitionsGraph(HttpServletRequest request, @RequestParam("tracker_id") Integer trackerId, Model model) throws AccessRightsException, PluginException {
UserDto user = ControllerUtils.getCurrentUser(request);
...
model.addAttribute("graphHtmlMarkup", result);
return "/bugs/tracker/workflowGraph.jsp";
}
}
}]
The first two methods are
Legacy REST API (v1) end-points.
The @ResponseView annotation will make sure, that the return value and any throw exceptions will be transformed into a proper JSON response.
The @RequestView annotation can be used, to transform the JSON request body into an internal codeBeamer entity.
Use @RequestBody get the raw JSON content.
The third method is an HTML
endpoint.
The return value is the (relative) path to the HTML
page or JSP
to return (View).
The page will show the data added to the specified Model.
Alternatively, you could return Model and View (JSP path) as a ModelAndView object.
EntityControllers
An EntityControllers is a special type of controller, that is responsible for a specific type of codeBeamer entity, e.g
- User
- Project
- Tracker
- Tracker Item
Typically an EntityController will convert the information from the received request into internal Data Transfer Objects (DTO) and then pass them on to the responsible EntityManager (see below).
E.g. the TrackerController shown partially above is the controller for Tracker entities, that are represented within codeBeamer as TrackerDto objects.
Data (Model) to be returned will be converted into an appropriate View, e.g.
EntityManagers
An EntityManager is a special type of component, that is responsible for the lifecyle (create, update, delete) and retrieving of codeBeamer entities of a specific type, e.g.
- UserManager is responsible for Users, User Groups and User Licenses,
- ProjectManager is responsible for Projects, Project Roles and Project Members,
- TrackerManager for Trackers and their configuration (Permissions, Fields, Workflow, ...)
The EntityManager is also responsible for applying proper entity access control and informing any
''Entity''Listeners about lifecyle events on their managed entity.
EntityDAO
An EntityDAO is a special type of component, that is responsible for the persistence (create, update, delete) and retrieving of codeBeamer entities of a specific type in the database.
Data Access Objects use MyBatis
to map Data Transfer Objects to Database objects (tables, rows, views, columns) and vice versa, and to create, update, delete and retrieve data in the database via JDBC
.
The EntityDAO is also responsible for maintaining the application level EntityCache, which is an EHCache
of the most recently used entities of that type.
Each EHCache
and also the EHCacheManager is itself a component.
Components
We have learned about some special types of components already above:
- EntityManagers
- EntityDAOs
- EntityCaches
There are a lot more:
- DefaultEntityListeners
- EntityContextMenuBuilders
- etc.
Custom Components
Some of the default codeBeamer controllers and managers allow to add custom add-ons/plug-ins to extend their basic functionality: